What is Scope
Without variable a program can only perform a small amount of tasks. The ability to store values and pull values out of variable gives a program state. Where we store variable and how we store it and all the rules related to it called scope.
Compiler Theory
Unlike the general notion that JS is interpreted language it’s compiled language. It is not compiled in advance. But, nevertheless, JS engine performs the same operation in more sophisticated way.
There are 3 steps in compilation :-
- Tokenising :- Breaking up string of characters inn large chunks of tokens, called tokens. For example var a = 2;. This program can be broken into 5 tokens. Which are var, a, =, 2, ;. Whitespace may or may not be persisted as token.
- Parsing :- Taking array of tokens and turning it into a tree to collectively present the grammatical structure of program is called parsing. Like in var a = 2;, we’ll first declare var as variable declaration with a child node a as identifier and another child element = as assignment operator. which will have child 2 as numerical identifier.
- Code generation :- Taking AST and generating an executable code is this process. It varies greatly depending on language and environment it’s running.
JS engine work is more complicated than just these 3 steps. In between parsing and cod generation there are steps to optimise the code to make execution faster and removal of redundant elements. For JS, compilation happens just before it’s execution.
The first thing compiler does is to perform the lexing to to break the program down into tokens and then parses it to abstract syntax tree. 2 distinct actions are taken for a variable assignment: first, compiler declare a variable in current scope and and in execution phase JS engine looks up to that variable in current scope and assign it to that variable.
Compiler look up for a variable :- So how does a compiler looks up for a variable in current scope? There are 2 ways to find the variable :- 1. LHS lookup and 2. RHS lookup.
LHS lookup is done when a variable appears on left side of assignment operator. RHS lookup is simply a look up of value for a variable.
For example :- in console.log(a), the reference to ‘a’ is RHS reference because nothing is being assigned to a here. In contrast, for var a = 2 compiler will depend on LHS lookup because here we are assigning 2 to variable a.
Consider this function to understand the LHS and RHS lookup better.

Here calling a function foo() requires RHS lookup. Compiler says to scope that ‘look for value of foo and give it to me’. And then compiler pass value 2 to function. Which is also RHS lookup. Then we are passing 2 as function argument. Inside the function argument a=2 is implied so value 2 is getting assigned to variable a. So inside the function, it’s LHS lookup for value of ‘a’. Then inside the function foo, when we are printing the value of a, resulting value of ‘a’ is being passed to log function. Looking up for value ‘a’ here is RHS lookup because we aren’t assigning anything. We are just printing the value of ‘a’. To print the value we need console object. Here also compiler will look for object console inside scope using RHS lookup and then a property lookup will occur to get the function log inside console object. And finally value of a will be printed in console. So, finally we saw here, 1 LHS lookup occurs and 4 RHS lookup occurs inside the scope.
This is how scope and compiler talk to each other.
Why does it matter if we call it LHS lookup or RHS lookup?
Because if we are looking for a variable using RHS lookup and couldn’t find it, it’ll throw a reference error. On contrast, if we are using LHS lookup and program isn’t running in strict mode then global scope will create a new variable of that same name and hand it over to engine saying that ‘there wasn’t one before, but I am helpful so created one for you.’
Building a metaphor
How does scope & nested scope works? We can think of it as a shopping mall. If we don’t find the item we were looking for on ground floor (current floor or currently executing scope) we go to second floor and then third and this way we go all the way to top floor. Now there are two scenario. First is either we find the item we were looking for or we will get reference error ‘Item not found’.

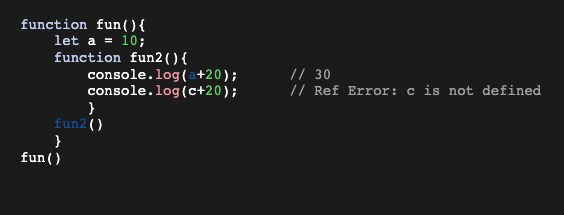
In this program fun2() will check for the value of ‘a’ in current scope i,e; within same function scope. It couldn’t find so it’ll to to next scope i,e; scope of parent function fun(). It’ll find the value of ‘a’ in fun(). It’ll get the value and print the result as 30. But if we’ll execute the next line. Since value of ‘c’ isn’t defined anywhere in the program it’ll keep looking for that value up to top most scope and will result in reference error as ‘c isn’t defined.’